Connecting Django Rest API with React and Angular: An In-Depth Guide
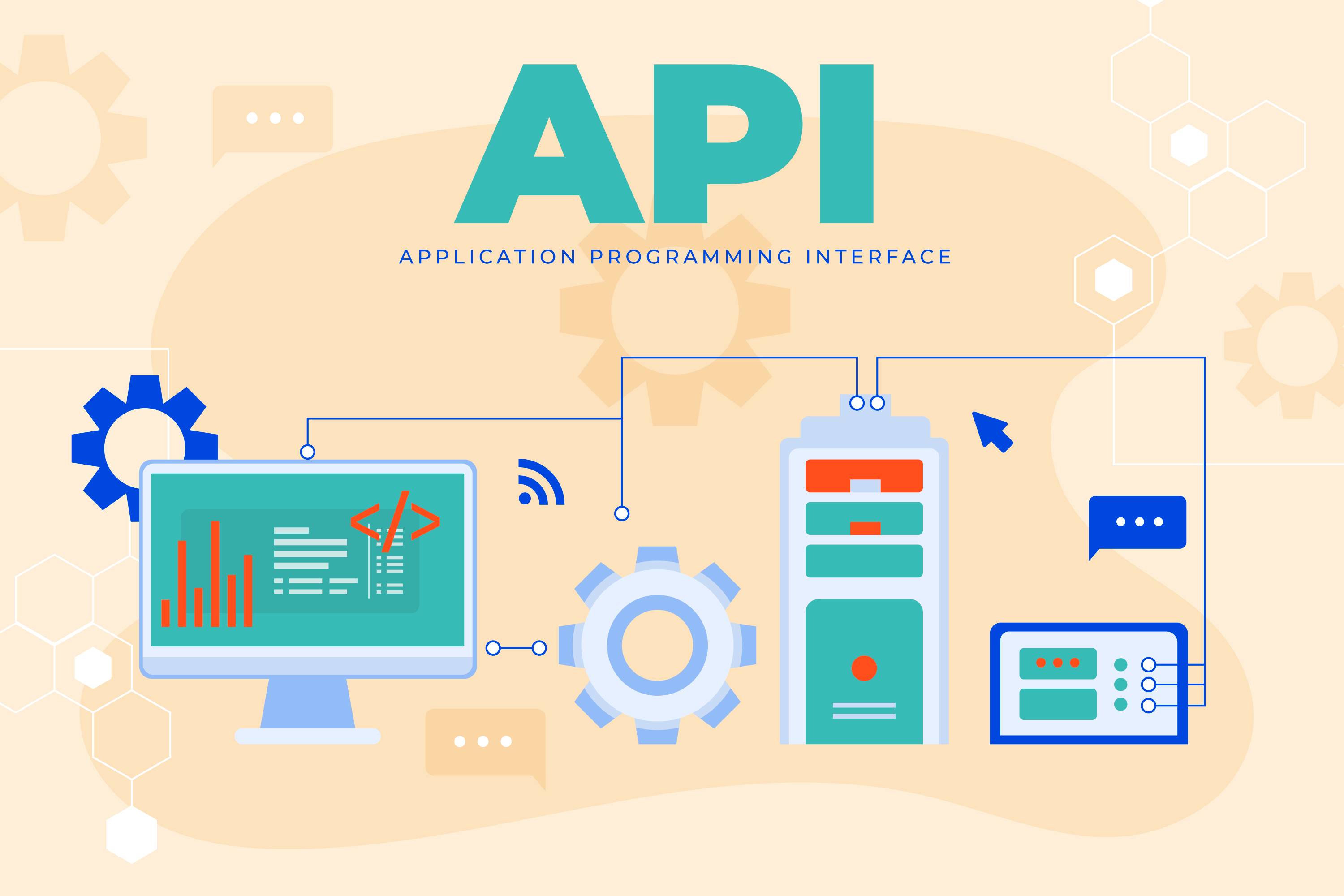
Connecting Django Rest API with React and Angular: An In-Depth Guide
Introduction:
Integrating Django Rest API with frontend frameworks like React and Angular can unlock the potential for building dynamic and interactive web applications. In this comprehensive guide, we will take a deep dive into the process of connecting a Django Rest API with React and Angular, covering everything from setup to data retrieval and form submissions. Let's explore each step in detail.
Step 1: Setting up the Django Rest API:
To begin, ensure that you have a Django Rest API set up. If you haven't already done so, create a new Django project and configure it to serve as a RESTful API using the Django Rest Framework (DRF). Define your models, serializers, and views to expose the desired data through API endpoints.
Step 2.1: Connecting Django Rest API with React:
When connecting Django Rest API with React, the process involves making HTTP requests from your React components to fetch data from the API and update the UI accordingly. Here's an in-depth breakdown of the steps involved:
1. Install required dependencies:
Create a new React project using Create React App or your preferred method.
Install Axios, a popular JavaScript library, for making HTTP requests.
Install React Router for handling client-side routing in your application.
2. Create API service:
Set up a separate file or folder in your React project to define functions for making API requests.
Import Axios and create functions that use Axios to send GET, POST, PUT, or DELETE requests to the Django Rest API.
You can define functions for specific API endpoints or create a more generic API service to handle multiple requests.
3. Fetching data:
Import the API service file into your React components that need to fetch data from the Django Rest API.
Use React's lifecycle methods or hooks (such as useEffect) to call the API service functions and retrieve the data.
Update the component state with the received data, and render it accordingly in your UI components.
4. Handling form submissions:
Create form components in React to allow users to submit data to the Django Rest API.
When the user submits the form, use Axios to send a POST or PUT request to the appropriate API endpoint, including the form data.
Handle the API response, update the component state if necessary, and provide appropriate feedback to the user.
Step 2.2: Connecting Django Rest API with Angular:
Similarly, connecting Django Rest API with Angular involves making HTTP requests to fetch data from the API and update the UI. Let's explore the process in detail:
1. Set up Angular project:
Create a new Angular project using the Angular CLI.
Configure any necessary dependencies for making HTTP requests.
2. Create a service:
Generate an Angular service using the Angular CLI to encapsulate the API logic.
Import the HttpClient module provided by Angular to make HTTP requests to the Django Rest API.
Define functions within the service to send GET, POST, PUT, or DELETE requests to the appropriate API endpoints.
3. Fetching data:
Import the API service into your Angular components that need to fetch data from the Django Rest API.
Use Angular's component lifecycle hooks (such as ngOnInit) to call the API service functions and retrieve the data.
Assign the received data to component variables and bind them to the UI for rendering.
4. Handling form submissions:
Create Angular forms using the FormBuilder module to allow users to submit data to the Django Rest API.
Use the HttpClient module to send POST or PUT requests to the appropriate API endpoints, including the form data.
Handle the API response, update the component state if necessary, and provide appropriate feedback to the user.
Conclusion:
By following the detailed steps outlined in this guide, you can successfully connect a Django Rest API with React and Angular, enabling seamless data exchange between the backend and frontend of your web applications. Remember to explore additional features and capabilities offered by Django, React, and Angular to enhance your development process and deliver outstanding user experiences. Happy coding!
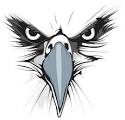