Creating an Image Slide with React and CSS Animations
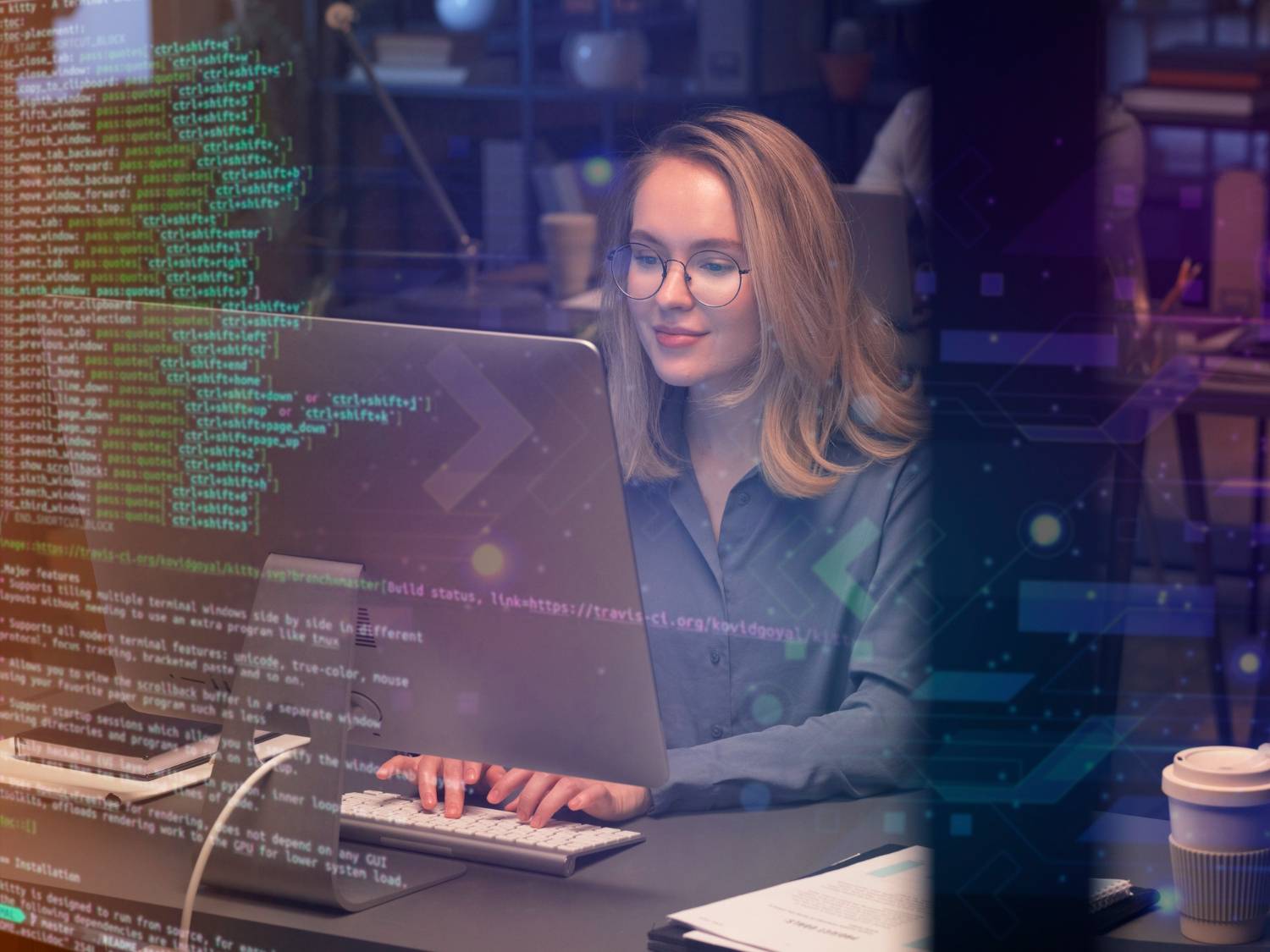
Title: Creating an Image Slide with React and CSS Animations
Here is a live demo of the image slider:
Introduction:
In this tutorial, we will walk you through the process of creating an image slide with React and CSS animations. The image slide will feature a sliding background effect and centered text for an engaging visual experience. Let's dive into the details and implementation steps.
Step 1: Setting up the HTML Structure and CSS Styles
To begin, let's define the HTML structure and CSS styles required for our image slide. Add the following code to your HTML file or React component:
```html
<!DOCTYPE html>
<html>
<head>
<style>
.wrapper {
width: 100vw;
height: 100vh;
overflow: hidden;
}
.sliding-background {
background: url("image1.jpg") repeat-y;
height: 200vh;
width: 100vw;
animation: slide 10s linear infinite;
}
@keyframes slide {
from {
transform: translateY(0);
}
to {
transform: translateY(-50%);
}
}
.sliding-background h1 {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
text-align: center;
color: white;
font-size: 2rem;
}
.text-container {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
text-align: center;
}
.text-container h1 {
color: white;
font-size: 2rem;
}
</style>
</head>
<body>
<div class="wrapper">
<div class="sliding-background">
<h1>Hello VenLax</h1>
</div>
<div class="text-container">
<h1>Your Text Goes Here</h1>
</div>
</div>
</body>
</html>
```
Step 2: Creating a React Component
Now, let's convert the HTML structure into a React component. Create a new file called `HomePage.js` and add the following code and above "css" code in external home.css file:
```jsx
import React from 'react';
import './home.css';
import externalImage from './image1.jpg';
class HomePage extends React.Component {
render() {
return (
<div className="wrapper">
<div
style={{
backgroundImage: `url(${externalImage})`,
}}
className="sliding-background"
>
<h1>Hello VenLax</h1>
</div>
<div className="text-container">
<h1>Your Text Goes Here</h1>
</div>
</div>
);
}
}
export default HomePage;
```
Step 3: Implementing the React Component in App.js
Finally, import and use the `HomePage` component in your `App.js` file:
```jsx
import React from 'react';
import './App.css';
import HomePage from './HomePage';
function App() {
return (
<div>
<HomePage />
</div>
);
}
export default App;
```
Conclusion:
In this tutorial, we walked through the process of creating an image slide with React and CSS animations. By setting up the HTML structure and CSS styles, and then converting it into a React component, we achieved the desired sliding background effect and centered text. You can now implement this image slide in your React applications to enhance visual appeal and engagement.
Remember to replace the `image1.jpg` URL with the actual URL or path to your desired image.
Feel free to experiment with different images, adjust the animation settings, and customize the text to suit your specific requirements.
Here is a live demo of the image slider:
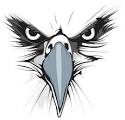